Op Art
BH: Only the last TIF is TIFfy, and I really think it should be a regular FYTD. Coloring the picture by hand is weird.
MF: BH has reviewing to do (see TG)
In this project, you will create tools to explore op art—a form of visual art using optical illusions.
Initially, the mouse is used to draw rectangles. Then when the user presses the space bar, the drawing phase ends and the mouse click becomes a paint bucket that fills bounded regions with paint. Here are two examples of phase 1 (drawing rectangles) and phase 2 (filling regions):
Example 1:
Example 2:
"U3-OpArt"
-
In the drawing phase (phase 1), you will be drawing many rectangles by clicking and dragging from corner to opposite corner, so it will be helpful to have an abstract data type (ADT) to manage the corner points.
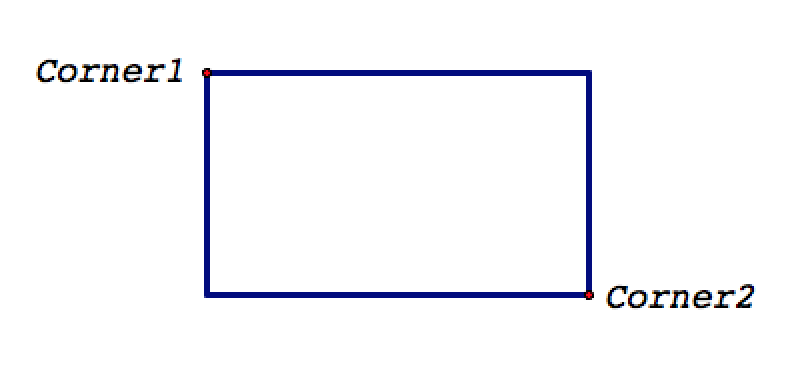
-
Create a constructor
corner
that will report a list of x and y coordinates of one corner.
-
Create two selectors
x of corner
and y of corner
that will report either the x or y values for an input corner.
-
Create two global variables to temporarily store the two points of each rectangle as it is drawn:
- current corner 1: to store the coordinates where the mouse is first clicked
- current corner 2: to store the coordinates where the mouse is moved as the rectangle is drawn (when the mouse is released, this value will be the final position of the second corner)
-
Now create a
rectangle
ADT for storing the two opposite corners of a single rectangle.

Like in the Animation Studio project on the previous page, the stage will have to be cleared and redrawn many times so that the user can see each new rectangle changing in size as it drawn. The program needs a way to store all of the rectangles that have already been drawn so that they can be redrawn along with the current rectangle after each stage clear.
- Create a global variable rectangle list to store the all the rectangles that have been drawn.
- Your program needs a way to know whether the user is finished drawing. Create a global variable drawing done? that will store a Boolean value (either
true
or false
) to keep track of the phase of the program (drawing or painting).
I don't think we need this. --MF, 9/10/18
It is initially false
but when the space bar is pressed—which concludes the drawing phase—it is set to true
.
-
The program also needs a way to draw a rectangle given its two defining corners. Create a
draw rectangle
block, and test that it correctly draws a rectangle with the input corners.
- Use the seven blocks and four variables you have created to create a program that:
- repeatedly collects rectangles until the user is finished phase 1 (drawing) by:
- collecting the coordinates of the first corner when the user starts to draw a rectangle
- repeatedly collecting the coordinates of the second corner and redrawing that rectangle and all previous rectangles until the user releases the mouse to finish the rectangle
- storing the final coordinates for each rectangle so they can be drawn again
- uses a keystroke (like space) to tell the computer to switch to phase 2 (painting) and then fills the clicked areas
Click for hints on creating this program.
- Which variables need to be initialized? Why?
- How can you detect the state of the mouse? How can you location the state of the mouse?
- You might want to use
and
to improve the efficiency and appearance of your program. The warp
block allows the drawing to happen quickly, and hide
hides the sprite.
I thought about keeping some version of this, but I think it is not needed given the above. --MF, 9/9/18
It is recommended that you first write down the algorithm detailing the sequence of events in the program and what should happen at each case before you build the actual code.
-
Create a version of your Op Art program that uses polygons.
-
Create a version of your Op Art program that uses circles.
-
Study the works of legendary Op Artists such as Victor Vasarely and Bridget Riley to get a feel for what is possible in this art form.
-
It is possible to fully automate the painting phase by using the following algorithm:
- for every pixel on the screen find out inside how many rectangles it is inside
- color each pixel black if the number is odd and white if the number is even (or vice versa)
To understand why this algorithm works, use paper and pencil to apply it to simple cases with few overlapping rectangles.
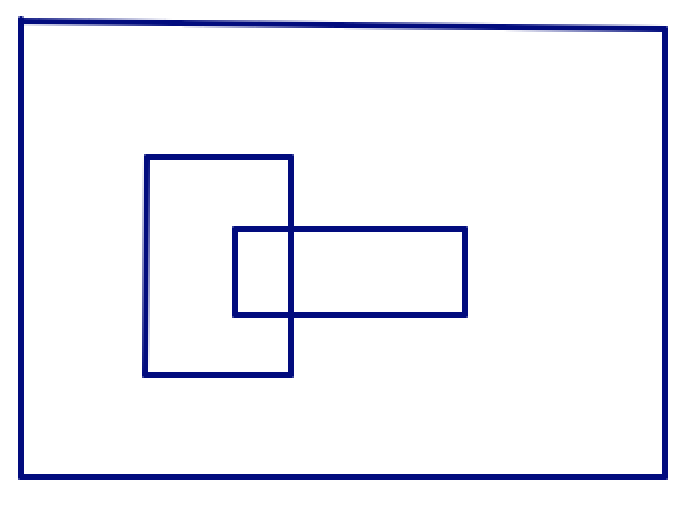
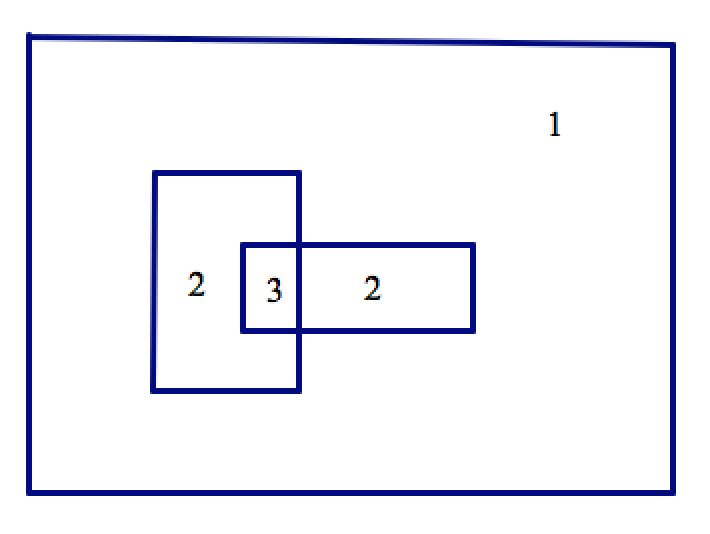