Managing List Data
This page does not belong in Unit 7! The stuff about sorting should be rewritten functionally, and the rest of it shouldn't be in the curriculum at all, imho. --bh
From michael (via GH):
- if discussing "index" variables, the word "position" should be used. The for each item is confusing because item is not an index. Using it on a word is OK, IMO, but there really needs to be an explicit comparison of the same operation between map / for each / explicit indexing if you want students to see the difference.
- @brianharvey: Your selection sort has a minor bug which filters out duplicate data. I don't know if there's a clean way to do it with a keep though, so a different version probably isn't as pretty.
Lists are essential to efficient data management. Lists can be counted, added to, removed from, selected from, searched in, and sorted. In this lesson, you will refine your Shopping List App, and you will explore common programming tasks related to lists: indexing, swapping, and sorting.
-
Review your "U2L2-ShoppingList" project from Shopping List App:
Contains
will be helpful here.
- If you didn't write code for the "Search" button, do it now. Clicking the "Search" button should prompt the user for a search term and then tell the user whether that item is already on the list (but not add the item). If you already wrote code for "Search" button, review your code and either try to improve it or help someone else create their own.
- Write a script for the "Delete Item" button that removes an item from the list if you haven't already.
-
Try re-writing your "Add Item" script a different way:
- either using
set
and in front of
- or using the
add
block
How do these two methods differ?
For each
and insert
will be helpful here.
-
Perhaps you coded your "Add Item" button to check to see if the item you want to add is already on the list so you don't create duplicates. Either way, create a "Clean Up List" button that removes any duplicate items from the list. Here are some instructions:
- Save this image to your computer:
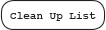
- Open and add a new sprite to your U3L1-ShoppingList project, call the sprite "Clean Up", and import the new button image on the Costumes tab for that sprite.
- Create a reporter
uniques
that takes a list as input and reports a list of each unique item in the original list.
Reporter blocks output (report) information based on the inputs, but they don't change the values stored in the input variable(s).
- Code the "Clean Up List" button to
set
the shopping list to a list of the unique items in shopping list.
Using an Index Variable
Part of what makes lists so powerful is that lists are ordered and each item in the list has an index number that you can use to refer to that particular item. But using an index variable to process a bunch of values is not specific to lists! Creating an index from scratch is fairly common in programming and worth getting comfortable with.
This block says each of the items in a list:
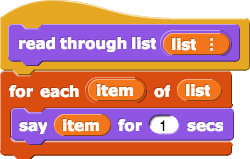
The block says all of the letters in a sentence:
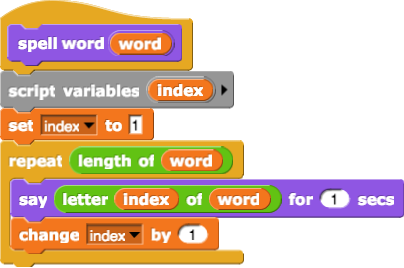
Since there is no for each letter
block that indexes strings of characters as if they were in a list, we must create an index variable and increase it by 1 (increment it) each time through the loop.
Read through each line of the spell word
script above, and discuss how it works.
-
Modify the script above to make the sprite say the following:
When writing a script, if you already have a similar script, then you can:
- Either duplicate the existing script and modify it for the new functionality
- Or create a more general script that can be used for both purposes.
- Every other letter in the word
- Every third letter
- All the letters in reverse order
- A range of letters (for example, letters 4-7) using either a
repeat until
or a repeat
block
DANGER BELOW: See Brian's note. We must actually create, not just imagine, the programs we ask kids to write. Problem b won't work, at least not as specified. Because of the
title of this section, I've included the whole section in the warning.
Swapping Variable Contents
Is this any different from what we already know about commands and reporters? What I think you really want to say here is this:
There are two very different styles of programming: functional programming, in which you mostly use reporters, and compute new values rather than modify values of variables that already exist; and imperative programming, in which you mostly use commands, and change the values of variables all the time. All else being equal, functional programming is better because it leads to fewer bugs and because it's much easier to parallelize. But sometimes it's more efficient or just plain easier to use imperative programming style. In Snap!, because of the way lists are implemented, it's important for efficiency reasons to use a consistent style for any particular list—either always use red command blocks to mutate the list, or always use reporters such as in front of
or keep
to compute new lists based on the old one.
But it's much too early to say that, which is why this whole page, if it belongs anywhere, belongs in Lab 4.
Sorting a List
(1) This animation goes way too fast to read. (2) Here's how I'd want them to think about selection sort:
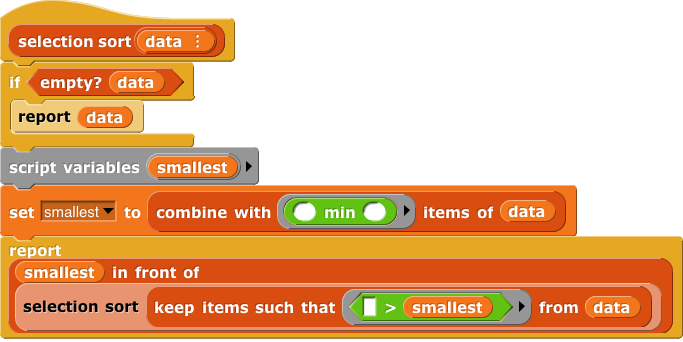
... but that's recursive, so if we had to do it in this unit, I guess I'd settle for
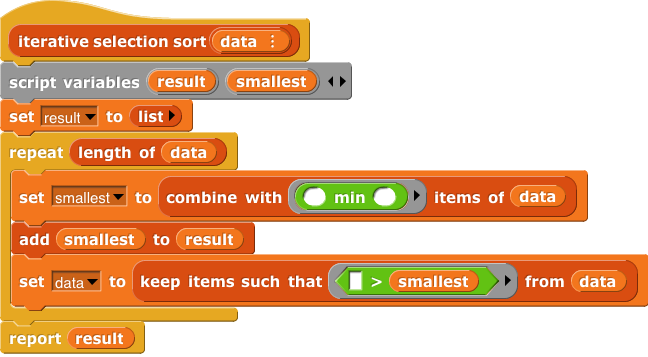
... but you can see how much uglier that is. So I'd rather do sorting in U7.
PG: Sorting is not appropriate here. It's complicated, the technique should be recursive, and it's not needed.
There are many different algorithms for sorting. Some are more efficient than others. Speed and memory matters a lot more when sorting with larger datasets. A simple sorting algorithm that works well with small datasets is the selection sort method shown right.
White is the original list. Yellow is the sorted list. Red is the current minimum. Blue is the current item being compared to the current minimum.
The double-headed arrow indicates a swap from one place in the list to another. The single-headed arrow indicates that the item that was there did not have to move.
Study the selection sort graphic at right. Discuss how this sorting algorithm works.
-
Imagine you already have a
lowest of items after?
predicate that takes an index number and a list as inputs and outputs (reports) the following:
true
if the list item at that index number is less than or equal to all of the items after it
false
if there is another item that is less than it after it.
Discuss how you could use your swap with
and lowest of items after?
blocks to build a selection sort script.
"U3L1-SelectionSort"
- Create a
lowest of items after?
predicate (as described above). Test it with a several items in a list of numbers.
Need script pic with result examples of block showing each output.
- Build a selection sort script.
Your swap with
and lowest of items after?
blocks will be useful here.